In this post, I'll show you how to create an ASP.NET Core API (.NET 5), how to versioning and add API documentation with Swagger. Also, you'll gonna learn how to apply this versioning to Swagger definitions, to don't specify all the time the version you want, and test your API quickly and intuitively.
Swagger describes REST APIs. It allows understanding the capabilities of a REST API.
In the last version of .NET (.NET 5), when creating a new .NET Core API project with Visual Studio, it is allowed to choose if swagger is wanted by checking "Enable OpenAPI support", which is checked by default.
Although, this swagger version is very basic. If we need to add additional features to Swagger, like Bearer Token or other custom parameters on the header we need to customize it.
A very common feature when developing APIs is versioning, and by default, it isn't mapped on Swagger automatically. By default, we need to specify it on a parameter when performing any request. Versioning is very helpful when we need to change the behavior of the API and we need to add some new features. Versioning will help us to not break the normal behavior of the users that are consuming our API.
To versioning a .NET Core API, we need to install the package "Microsoft.AspNetCore.Mvc.Versioning". You can install it by Package Manager or running the command "Install-Package Microsoft.AspNetCore.Mvc.Versioning". After the installation, you'll be able to use the attribute "ApiVersion" in the API's controllers.
In order to help the versioning process, we're going to create a base class (ApiControllerBase). The file content is the following:
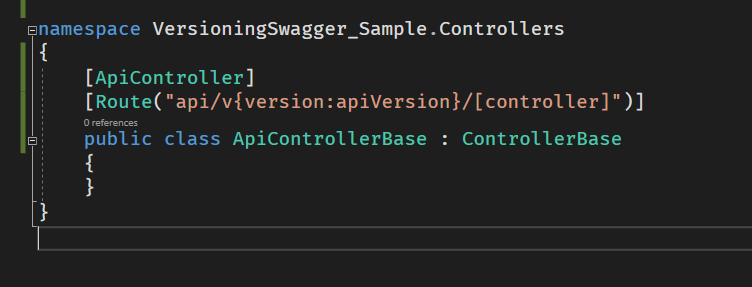
The class inherits the ControllerBase and maps the for example the following URL structure: https://localhost:5001/api/version_of_api/name_of_controller
Now, in the controller, we can remove the default attributes, add the ApiVersion attribute, and inherits the controller from our ApiControllerBase.
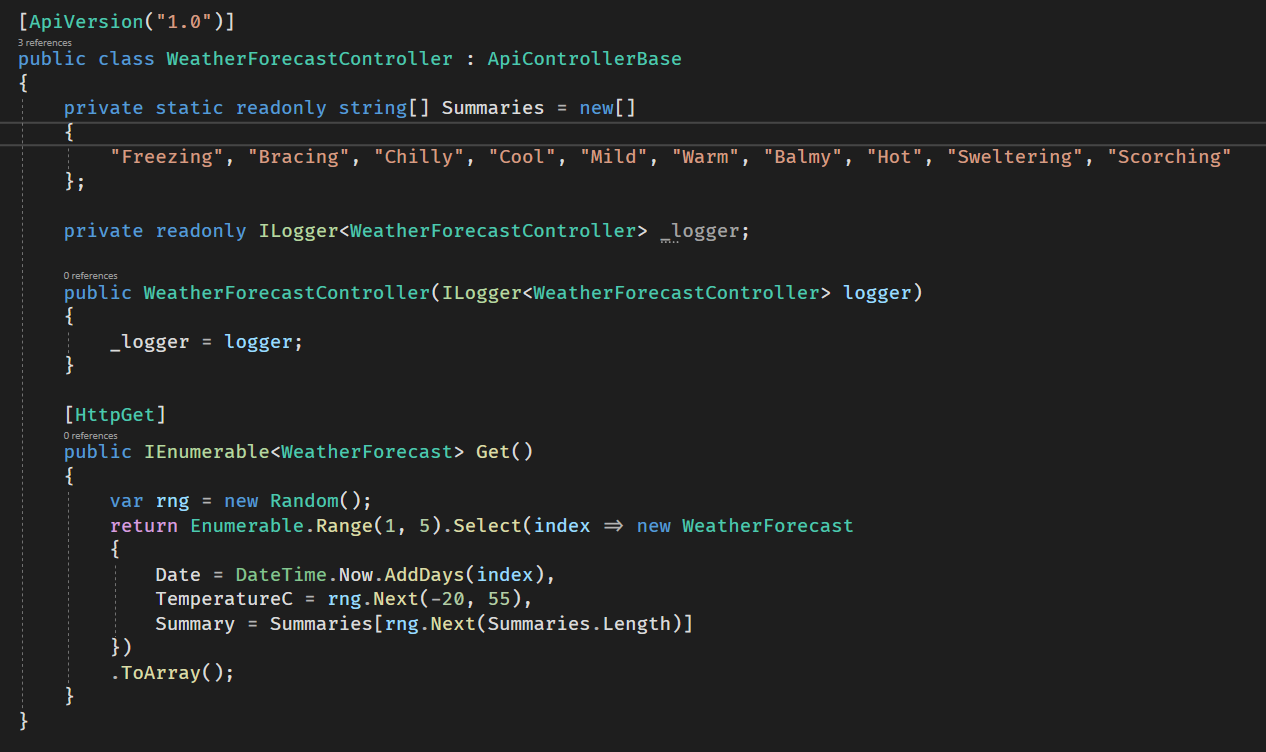
If you run the application and try to make a request, swagger is already requesting the version.
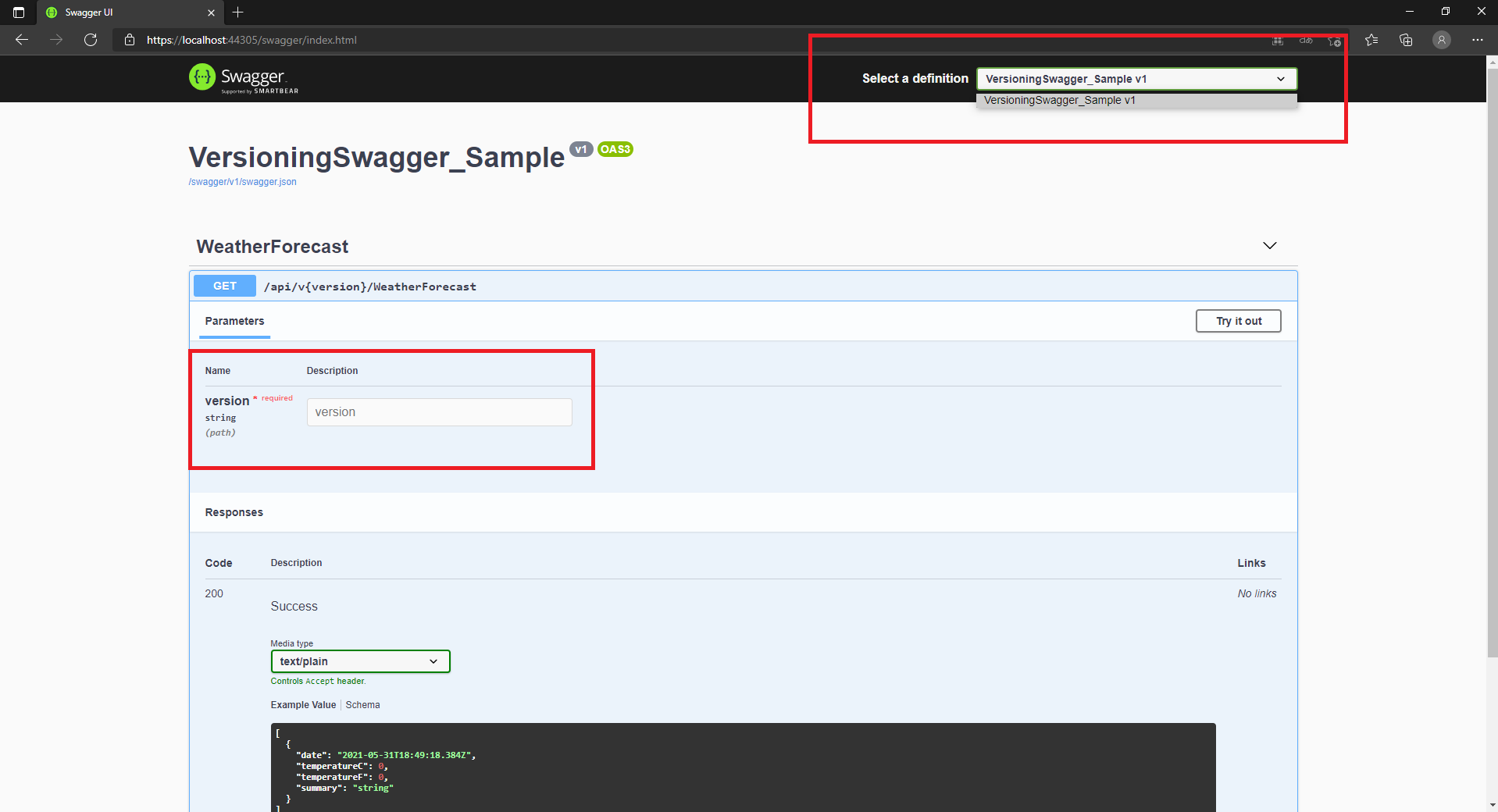
This is not perfect, because we have always to specify the version instead of selecting the definition on the header of the page.
To improve this, install the package "Microsoft.AspNetCore.Mvc.Versioning.ApiExplorer".
After the installation, update the content of the startup file like the following:
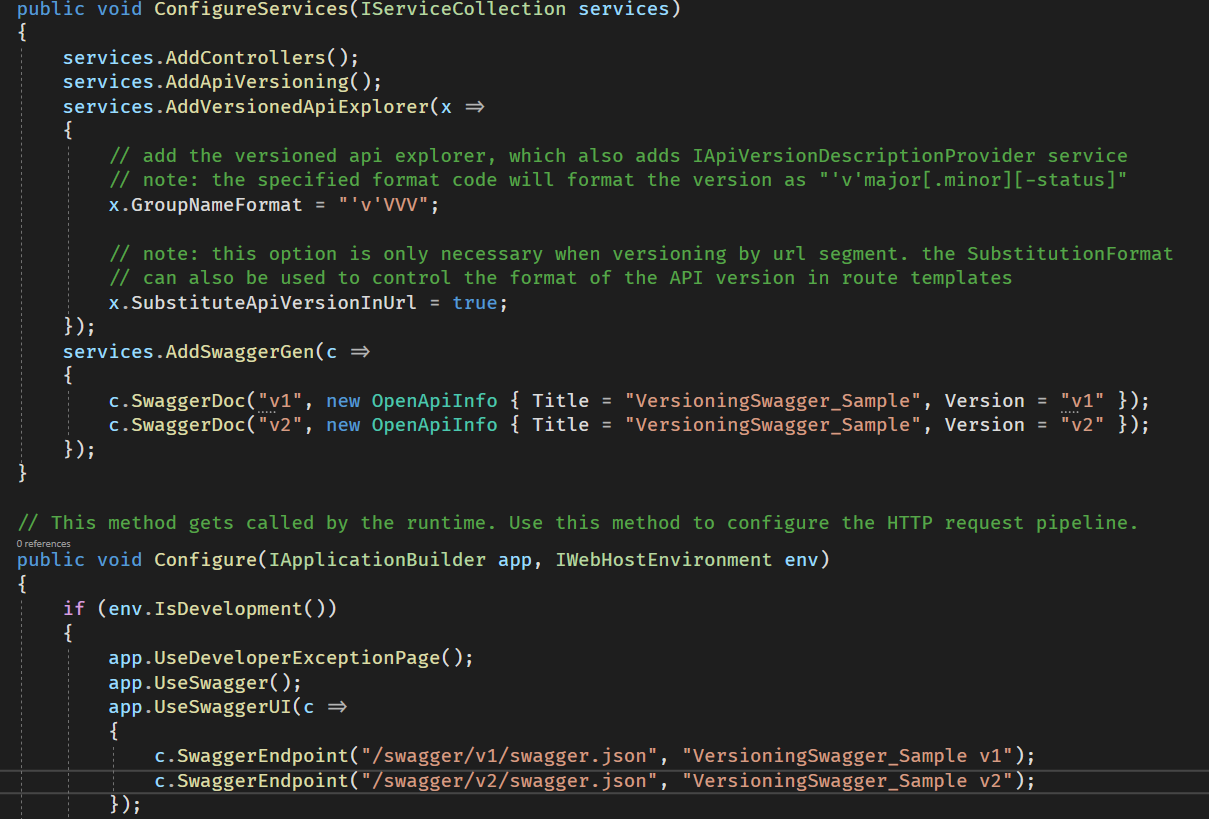
For a better understanding, create a copy of the WeatherForecastController, rename it to "WeatherForecastV2Controller" and change de ApiVersion to "2.0".
Run the application. Now, we can choose the version on the definition automatically!
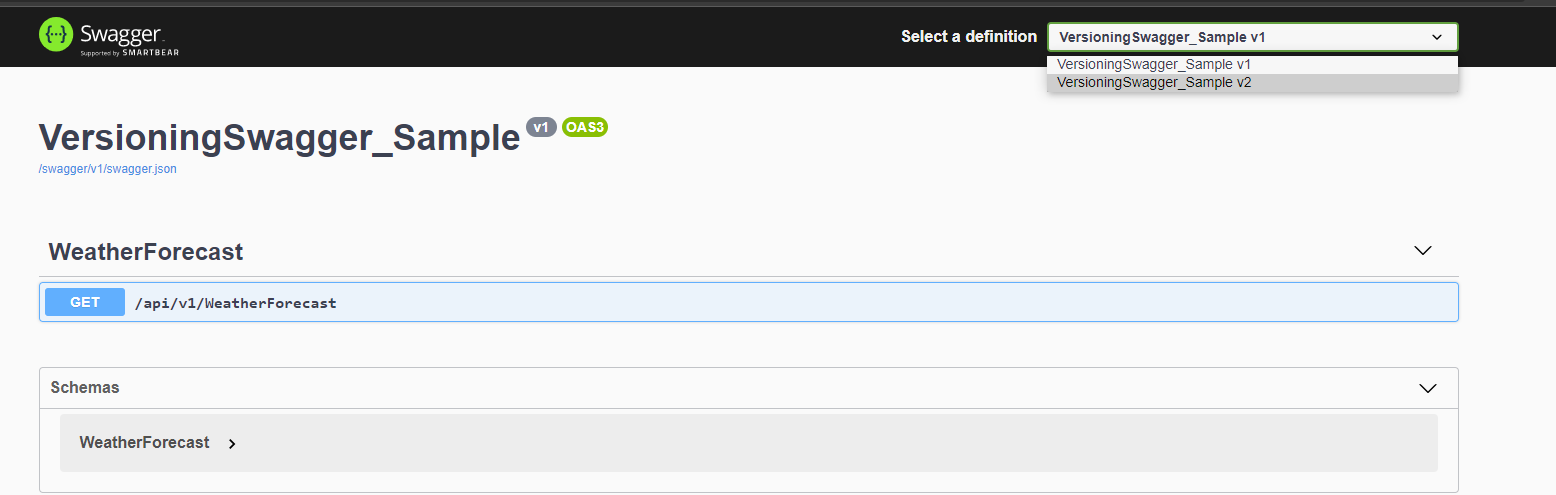
To make this post simple, I inserted the definitions manually. We can improve this, and add new version definitions to swagger automatically. If you want to improve, send me a message.
For more information about Swagger, visit the Microsoft official documentation.
You can find the repo on my GitHub.
Hope you enjoyed it and learned something new!
Header image from Unsplash